When working with Python, one common requirement is to create a directory if it doesn’t already exist. Knowing how to Python create a directory if it doesn’t exist is an important skill to have. Whether you’re developing an application that needs a specific directory structure or writing a script to manage files, being able to create directories dynamically is crucial.
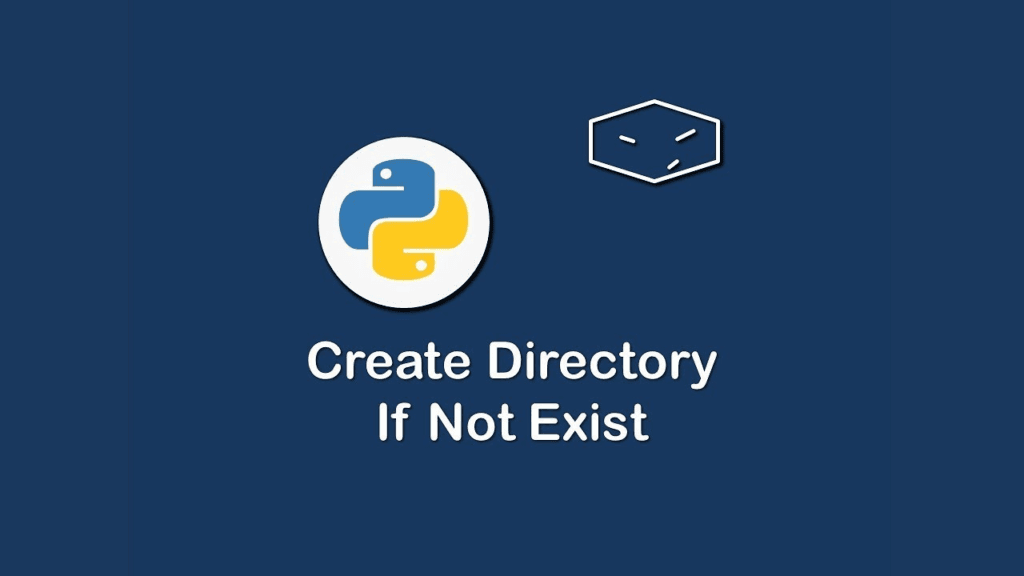
In this article, we will explore different methods and techniques to achieve this task. By understanding how to Python create a directory if it doesn’t exist, you will have the necessary knowledge to handle file management effectively and ensure that your code runs smoothly in various scenarios. So, let’s dive into the topic of “How to Python create a directory if it doesn’t exist” and learn the approaches to accomplish this task efficiently.
Understanding the Challenge: Conditional Directory Creation
Before we delve into the solutions, let’s gain a clear understanding of the problem at hand. We are faced with the task of creating a directory programmatically, but with a condition. If the directory already exists, we don’t want to create it again. This conditional behavior ensures that our code remains efficient and doesn’t create redundant directories.
The Significance of Conditional Directory Creation
Conditional directory creation plays a vital role in various programming tasks. It allows us to handle scenarios where we need to store data in specific locations without worrying about overwriting existing directories. Additionally, it simplifies the process of managing and organizing files dynamically.
Approach 1: Using os.makedirs()
The os.makedirs() Function:
Python’s built-in os module provides a convenient function called makedirs() that allows us to create directories recursively. By utilizing this function along with conditional checks, we can create directories only if they don’t already exist.
Implementation and Example:
To create a directory using os.makedirs(), we can simply pass the desired directory path as an argument. However, to ensure conditional creation, we need to perform a check using the os.path.exists() function before calling makedirs().
Here’s an example illustrating the implementation:
python
import os def create_directory_if_not_exists(directory_path): if not os.path.exists(directory_path): os.makedirs(directory_path) print("Directory created successfully!") else: print("Directory already exists.") # Usage: create_directory_if_not_exists("/path/to/my/directory")
In this example, we first check if the directory path already exists using os.path.exists(). If it doesn’t exist, we call os.makedirs() to create the directory recursively. Otherwise, we display a message indicating that the directory already exists.
Approach 2: Using pathlib.Path.mkdir()
The pathlib.Path.mkdir() Method:
Python’s pathlib module offers an object-oriented approach to file system path manipulation. With the Path class and its mkdir() method, we can create directories conditionally without the need for additional conditional checks.
Implementation and Example:
To create a directory using pathlib.Path.mkdir(), we first create a Path object with the desired directory path. Then, we call the mkdir() method, specifying the exist_ok=True parameter to ensure that the directory is created only if it doesn’t already exist.
Here’s an example illustrating the implementation:
python
from pathlib import Path def create_directory_if_not_exists(directory_path): directory = Path(directory_path) directory.mkdir(exist_ok=True) if directory.exists(): print("Directory created successfully!") else: print("Directory already exists.") # Usage: create_directory_if_not_exists("/path/to/my/directory")
In this example, we create a Path object with the directory path and call mkdir() with the exist_ok=True parameter. This ensures that the directory is created only if it doesn’t already exist. We then perform a check using the exists() method to confirm whether the directory was successfully created or if it already existed.
Approach 3: Using try-except Block
The try-except Block:
Another approach to conditionally create a directory in Python is by utilizing a try-except block. We can attempt to create the directory using the os.makedirs() function within a try block. If the directory already exists, an exception will be raised, which we can catch and handle accordingly.
Implementation and Example:
Here’s an example showcasing the implementation of the try-except approach:
python
import os def create_directory_if_not_exists(directory_path): try: os.makedirs(directory_path) print("Directory created successfully!") except FileExistsError: print("Directory already exists.") # Usage: create_directory_if_not_exists("/path/to/my/directory")
In this example, we use the try block to call os.makedirs() and attempt to create the directory. If the directory already exists, a FileExistsError exception will be raised. We catch this exception in the except block and handle it by displaying a message indicating that the directory already exists.
Conclusion:
Efficient file management is a cornerstone of successful programming endeavors. By mastering the art of conditional directory creation, we can simplify our code and ensure optimal file organization.
Throughout this journey, we explored three different approaches to conditionally create directories in Python. The os.makedirs() function, when combined with conditional checks, provides a straightforward solution. The pathlib.Path.mkdir() method offers an object-oriented alternative, eliminating the need for additional checks. Lastly, the try-except block allows us to catch exceptions and handle them gracefully.
Remember to choose the approach that aligns best with your coding style and project requirements. Whether it’s the simplicity of os.makedirs(), the elegance of pathlib.Path.mkdir(), or the versatility of the try-except block, each approach offers a unique perspective on conditional directory creation. So go forth, empower your file management capabilities, and streamline your code with the ability to create directories dynamically, only when needed. Embrace the beauty of conditional logic, and conquer the world of file manipulation with confidence and efficiency.